4 User Guide - Reference Documentation
Authors: Martin Schimak
Version: 0.5.0
Table of Contents
4 User Guide
After having installed the plugin you can immediately start to use the camunda process engine.4.1 Create a process definition
You can create a BPMN 2.0 process definition by issueing the following command:grails create-process [name]
| Created file grails-app/processes/org/camunda/my/TestProcess.bpmn | Created file test/integration/org/camunda/my/TestProcessSpec.groovy
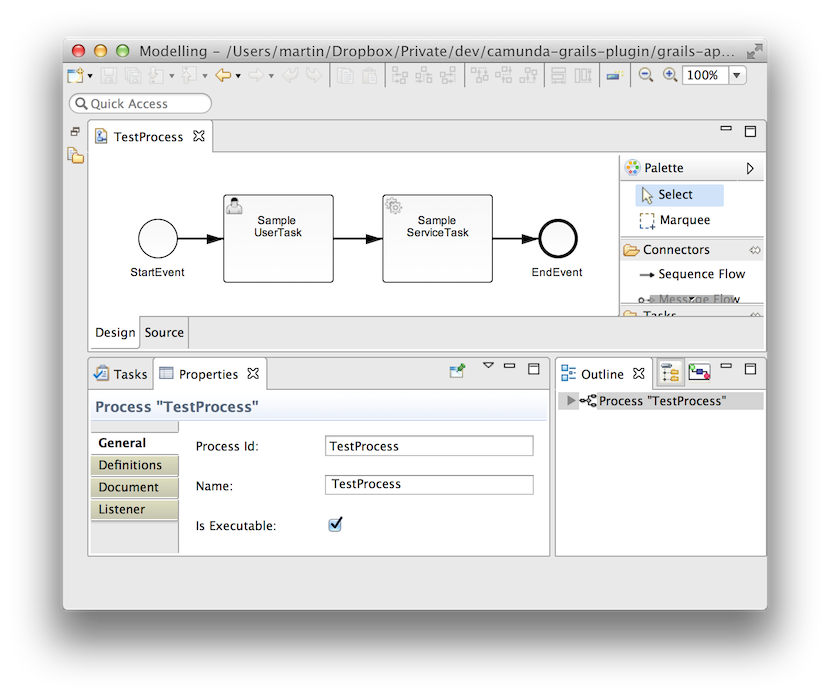
[...] given: "a new instance of TestProcess" runtimeService.startProcessInstanceByKey("TestProcess")when: "completing the user task" def task = taskService.createTaskQuery().singleResult() taskService.complete(task.id)then: "the service method defined for the subsequent service task was called exactly once" 1 * sampleTestProcessService.serviceMethod(_ as Execution) [...]
4.2 Inject camunda API Services
You can easily use the process engine internally in your application. Just inject the camunda process engine API services into your grails artifacts (like controllers, services, etc). The following services can be found in the package org.camunda.bpm.engine and are made available under their 'bean style' class names, as shown here in alphabetical order:import org.camunda.bpm.engine.*
…
AuthorizationService authorizationService
FormService formService
HistoryService historyService
IdentityService identityService
ManagementService managementService
RepositoryService repositoryService
RuntimeService runtimeService
TaskService taskService
CaseService caseService
FilterService filterService
Note that many of these bean names are quite generic (like 'taskService', 'managementService' etc) and could easily clash with names you want to use in your application for other purposes - even more so when having in mind the Grails naming convention to use *Service as a name pattern for Grails services. On the other hand typical camunda users are used to the service names shown above and will expect to use these services via their established names. Therefore the plugin provides these services via those names shown above. However, in order to avoid potential name conflicts with grails services pre-existing in your project, the plugin also allows to change these default bean names via configuration.In addition to the camunda API services, the ProcessEngine itself can be used as an injectable dependency, too:
import org.camunda.bpm.engine.*
…
ProcessEngine processEngine
4.3 Develop a camunda BPM tasklist application
Using the camunda BPM tasklist with 'embedded' forms as described in this section, will just work with a 'shared' process engine deployment scenario.
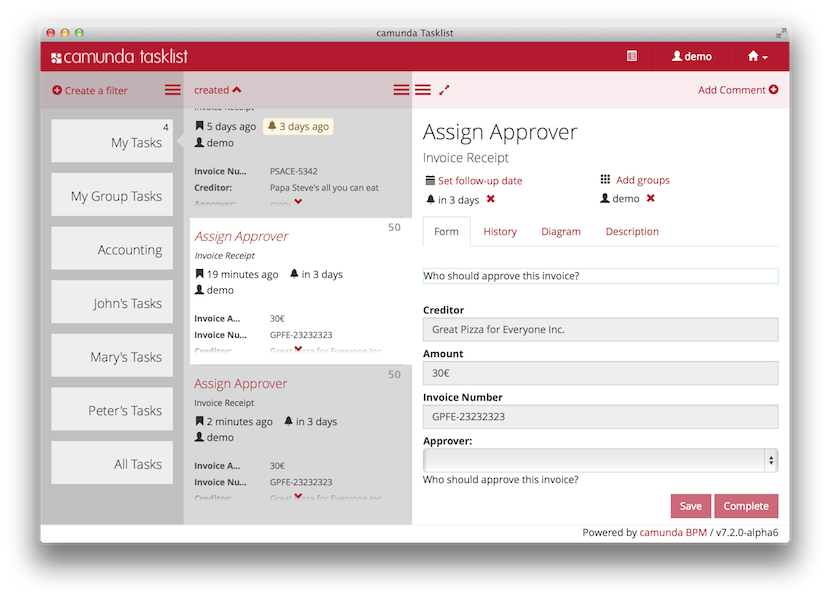
/grails-app/views/test/sampleForm.gsp
<userTask id="UserTask_1" name="Sample UserTask" camunda:formKey="embedded:app:camunda/test/sampleForm"/>
<startEvent id="StartEvent_1" name="Sample StartEvent" camunda:formKey="embedded:app:camunda/test/sampleForm"/>
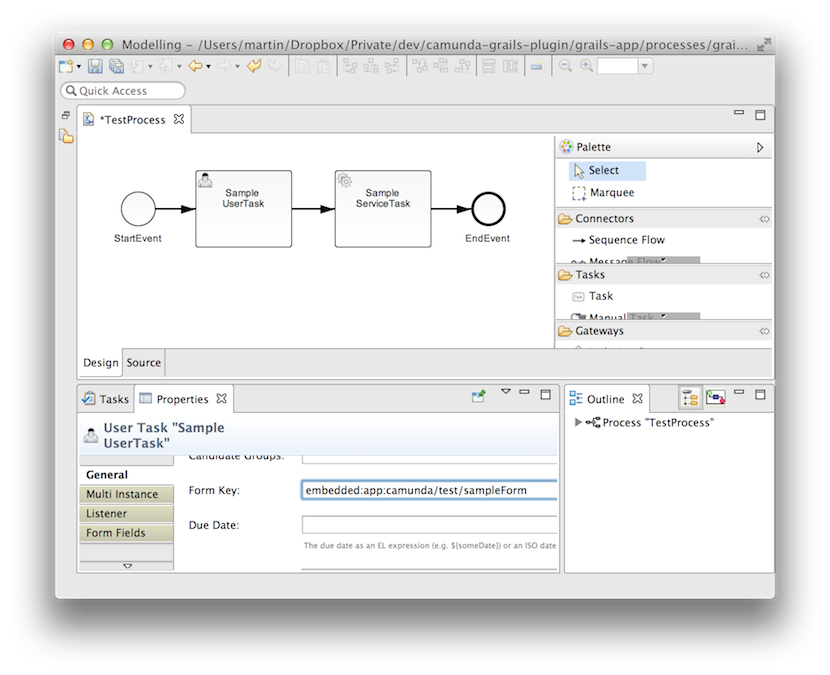
Make sure that the body of your gsp just contains a single form element and that the html follows the conventions for camunda embedded forms. Of course you are free to dynamically generate that html via gsp mechanisms. To learn more about camunda embedded forms, you may want to look at the raw html code of the sample form provided with camunda Grails Plugin or consult camunda's embedded-task-forms docs.
4.4 Redirect camunda BPM tasklist to your own grails application
Of course, you are also free to use your own controller actions to deliver forms, be it for embedded purposes...camunda:formKey="embedded:app:forms/index"
camunda:formKey="app:forms/index"
taskId
url parameter to look up the task as well
as with a callbackUrl
parameter to redirect back to the task list.